Mastering Swift Property Observer CRUD: A Simple Guide to Operations

Swift property observer CRUD is a powerful feature that enhances how developers manage data in their applications. By utilizing property observers, programmers can monitor changes to properties automatically, allowing for smoother and more responsive apps. This is especially useful for tasks like updating user interfaces in real-time without extensive coding.
At Trust Blogs, we focus on delivering easy-to-understand content for tech enthusiasts and developers. Our goal is to simplify complex concepts like Swift property observers so that everyone can grasp their importance. By integrating these techniques, developers can create more interactive and efficient applications, making their coding journey more enjoyable.
What is Swift Property Observer CRUD?
Swift property observer CRUD is a special feature in Swift programming that helps you monitor changes to variables. When a variable’s value is modified, property observers allow you to respond automatically. This makes CRUD (Create, Read, Update, Delete) operations more efficient and smoother to manage.
For example, when you update data in an app, property observers can track these changes and trigger an action. Whether it’s saving the new data or handling an error, this function is a simple yet powerful tool. It helps developers keep their apps more dynamic and responsive without writing extra code.
With CRUD operations being the heart of data management, Swift property observer CRUD ensures everything is well-maintained. It provides real-time feedback when data is created, updated, or deleted. This gives developers more control over their app’s behavior.
Why You Should Use Swift Property Observers in CRUD Operations
Swift property observers are very helpful in CRUD operations. When you create or update data, property observers notify you instantly about any changes. This makes your code cleaner and more efficient because you don’t have to manually check every change.
If you’re working with databases or any data-heavy projects, these observers can simplify your work. You can easily perform CRUD tasks without worrying about missing any updates. It makes tracking data flow within your app a much easier process.
In addition, using Swift property observer CRUD improves the overall performance of your app. It ensures that only the necessary code is triggered when changes occur, which saves time and system resources. This is important, especially in apps that manage a lot of data.
How Swift Property Observer CRUD Makes Your App More Dynamic
With Swift property observer CRUD, your app becomes more dynamic because it responds automatically to data changes. This means you don’t have to manually refresh data or call functions every time a variable is updated. The observers handle it all behind the scenes.
For instance, if you’re updating user profiles or shopping carts in an app, Swift property observer CRUD makes the process smoother. It allows the app to instantly reflect the changes without any delays. This is especially useful in real-time applications where data needs to be updated frequently.
By using Swift property observer CRUD, developers can create apps that feel more alive and interactive. It eliminates the need for constant manual intervention, making both development and user experience much better.
Step-by-Step Guide to Implementing Swift Property Observer CRUD
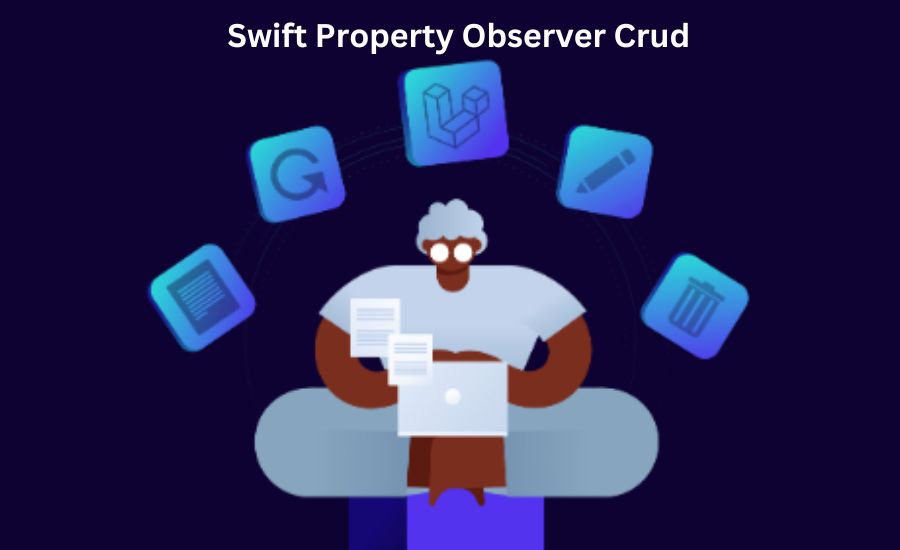
Implementing Swift property observer CRUD is quite simple. First, declare a variable and attach the property observers willSet
and didSet
. These observers track changes before and after the variable is modified. This is where you can trigger CRUD actions based on the new or old values.
For example, if you want to update the UI after a change, use the didSet
observer. It will run the code right after the value is changed. Similarly, you can use willSet
to prepare for an upcoming change, like saving the current state before the new value is assigned.
By following these steps, you can easily integrate Swift property observer CRUD into your project. It’s a straightforward process that helps keep your code organized and efficient. You don’t need to constantly worry about manually tracking every change in your data.
Understanding the Basics of Swift Property Observers
Property observers in Swift help you observe and respond to changes in a variable’s value. There are two types of observers: willSet
and didSet
. The willSet
observer runs before the new value is set, while didSet
runs after the value has changed.
This feature is especially useful in CRUD operations, where data changes frequently. With Swift property observer CRUD, you can handle these updates seamlessly. For example, when data is created or deleted, observers can trigger actions like saving the changes or displaying alerts.
Understanding these basics will help you use Swift property observers effectively in your apps. It makes managing data much easier and ensures that your app stays updated with the latest changes.
Create, Read, Update, and Delete with Swift Property Observer CRUD
When working with CRUD operations, Swift property observers can be your best friend. For instance, you can use didSet
to automatically save data after it’s updated, or willSet
to prepare the system before deleting data. This makes CRUD operations smoother and less error-prone.
In many apps, data is constantly created, read, updated, or deleted. Swift property observer CRUD simplifies these operations by tracking changes automatically. This not only saves time but also ensures that your data management is efficient and accurate.
By integrating Swift property observer CRUD into your app, you can automate a lot of tasks. Whether it’s updating the database or refreshing the UI, the process becomes seamless. This helps keep your app functioning at its best.
How to Track Changes in Your App Using Swift Property Observer CRUD
Tracking changes in your app is essential, especially when working with large amounts of data. With Swift property observer CRUD, you can easily track any changes in your variables. This helps you ensure that your app is always up-to-date with the latest information.
For example, in an e-commerce app, you can track when a product’s price changes or when items are added to the cart. Swift property observers allow you to respond to these changes in real-time, ensuring a smooth user experience.
Using property observers is a great way to make your app more interactive. It allows you to handle changes efficiently without adding too much extra code. With Swift property observer CRUD, change-tracking becomes a breeze.
Top Benefits of Using Swift Property Observer CRUD in Your Code
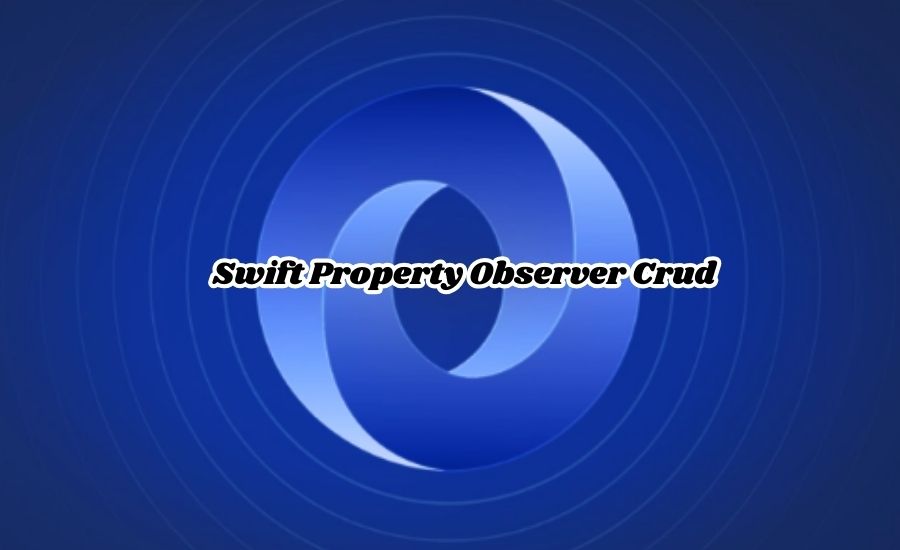
Swift property observer CRUD comes with many benefits.
- First, it reduces the need for extra code. Since observers automatically track changes, you don’t have to write complex logic to handle every update. This makes your code simpler and easier to manage.
- Second, it improves app performance. By only triggering actions when necessary, Swift property observers help reduce the workload on your app. This ensures that your app runs smoothly without consuming too many resources.
- Lastly, it makes debugging easier. With property observers, you can see exactly when and where changes happen in your data. This helps you quickly identify and fix any issues in your CRUD operations, saving you time and effort.
Common Mistakes to Avoid in Swift Property Observer CRUD
When working with Swift property observer CRUD, there are a few common mistakes to avoid. One of the biggest mistakes is overusing property observers. While they are powerful, using them too often can slow down your app, especially in large projects.
Another mistake is not understanding when to use willSet
and didSet
. Make sure you use them in the right situations. For example, willSet
is best for preparing for a change, while didSet
should be used to handle the result of a change.
By avoiding these mistakes, you can ensure that your Swift property observer CRUD code runs smoothly. It’s important to use observers wisely and only when necessary to keep your app optimized.
Swift Property Observer CRUD: A Beginner’s Friendly Example
Let’s say you have a shopping cart in your app. Every time a user adds or removes an item, you want to update the total price. With Swift property observer CRUD, you can easily track changes to the cart and recalculate the total whenever the contents change.
You can use the didSet
observer to run a function that updates the price each time an item is added or removed. This makes your app more responsive, and users can see the updated total in real-time without refreshing the page.
This simple example shows how useful Swift property observer CRUD can be in real-life applications. It helps you manage data changes easily, making your app more user-friendly.
How to Optimize Your App with Swift Property Observer CRUD
To optimize your app, Swift property observer CRUD can be a valuable tool. It allows you to respond to data changes without having to manually check for updates. This reduces the need for repetitive code and keeps your app running efficiently.
For example, you can optimize a news app by using property observers to track when new articles are added. Instead of reloading the entire app, the observer can simply update the relevant section of the UI. This saves both time and system resources.
By using Swift property observer CRUD, you can ensure that your app stays fast and responsive. It streamlines data handling, making your app more efficient without compromising performance.
Swift Property Observer CRUD: Best Practices You Should Follow
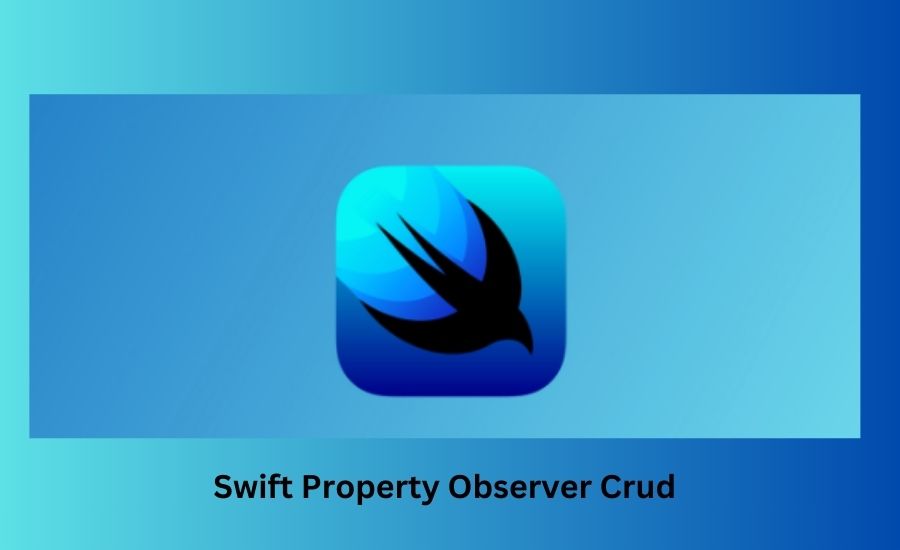
When using Swift property observer CRUD, it’s important to follow best practices. First, avoid overloading your observers with too many tasks. Keep their functions simple and focused to avoid slowing down your app.
Second, always test your observers thoroughly. Make sure they trigger the correct actions when data is created, updated, or deleted. This will help prevent bugs and ensure that your CRUD operations work smoothly.
Lastly, use property observers only when necessary. While they are a great tool, not every variable needs to be monitored. By following these best practices, you can use Swift property observer CRUD effectively in your projects.
Need To Know: How-Atp-On-Hand-Refresh-d365-Finance-And-Operations
Debugging Tips for Swift Property Observer CRUD
Debugging Swift property observer CRUD can be tricky if you’re not careful. One tip is to use print statements within your willSet
and didSet
observers. This lets you see exactly when and where a change occurs, helping you track down any issues.
Another helpful tip is to isolate the observer in a separate function. This way, you can test it independently from the rest of your code. If something goes wrong, it’s easier to find the root cause without affecting the rest of your app.
By following these debugging tips, you can ensure that your Swift property observer CRUD code works correctly. It will help you identify problems quickly and fix them before they become bigger issues.
Swift Property Observer CRUD: How It Improves Code Efficiency
Using Swift property observer CRUD is a smart way to make your code more efficient. By automating the tracking of data changes, you reduce the need for manual checks. This makes your code cleaner and easier to read, allowing you to focus on more important tasks.
In addition, property observers streamline CRUD operations. Instead of writing separate functions to handle every data update, you can use observers to trigger actions automatically. This saves time and reduces the chance of errors in your code.
Overall, Swift property observer CRUD helps create efficient, organized, and responsive applications. By leveraging this feature, developers can build better apps that respond to data changes seamlessly.
Real-Life Use Cases of Swift Property Observer CRUD in iOS Development
In iOS development, Swift property observer CRUD is widely used in many apps. For instance, in social media apps, property observers can track changes in user profiles or messages. This allows users to see updates in real-time, enhancing the overall experience.
Another use case is in financial apps, where property observers can monitor account balances. Whenever a transaction occurs, observers can automatically update the balance and notify users. This ensures that users have the latest information at their fingertips.
Overall, Swift property observer CRUD has many practical applications in real-life iOS development. It helps create interactive and user-friendly apps by automatically managing data changes efficiently.
Conclusion
In conclusion, Swift property observer CRUD is a powerful tool for app developers. It helps track changes in data easily and automatically. This means developers can create apps that respond quickly to user actions without writing too much code. By using property observers, you can keep your app running smoothly and efficiently.
Using Swift property observer CRUD not only makes coding easier, but it also improves the experience for users. They get instant updates, making apps feel more lively and interactive. So, whether you are building a game or a shopping app, remember that property observers can make your app better and more fun to use!
Do You Know: Dacac24
FAQs
Q: What is Swift property observer CRUD?
A: Swift property observer CRUD is a feature in Swift programming that helps developers monitor changes to variables automatically, making data management easier and more efficient.
Q: How do property observers work in Swift?
A: Property observers use two types of functions: willSet
, which runs before a variable’s value changes, and didSet
, which runs after the value has been updated. These functions allow you to respond to changes in real time.
Q: Why should I use property observers in my app?
A: Using property observers simplifies your code and automates data handling, making your app more responsive and improving performance without requiring extra coding.
Q: Can property observers help with real-time updates in my app?
A: Yes! Property observers enable real-time updates, allowing your app to instantly reflect any changes in data, which enhances user experience and interaction.
Q: Are there any common mistakes to avoid when using property observers?
A: Common mistakes include overloading observers with too many tasks and not understanding when to use willSet
versus didSet
. Keeping your code simple helps avoid issues.
Q: How can I debug property observers effectively?
A: You can debug property observers by adding print statements in your willSet
and didSet
functions to track changes. This helps identify issues quickly and ensures everything works correctly.
Q: What are some real-life examples of using Swift property observer CRUD?
A: Real-life examples include tracking changes in social media profiles, updating shopping cart totals in e-commerce apps, and monitoring account balances in banking applications.